本ページでは、Python のグラフ作成ライブラリの matplotlib で折れ線グラフ を描く方法について紹介します。
matplotlibとは?
matplotlib (マットプロットリブ) は、Python のグラフ作成ライブラリで、各種グラフを作成・可視化することができます。
matplotlibの読み込み
import matplotlib.pyplot as plt import numpy as np
matplotlibを使うために、matplotlib.pyplot を plt という名前で読み込みましょう。
また、numpyライブラリもインポートしています。
matplotlibで折れ線グラフを作成
matplotlibでグラフを作成するには、基本的にデータ生成して描画を行えばOKです。
#データ生成 x = np.arange(0, 5, 0.1); y = np.sin(x) #描画 plt.plot(x, y) #プロットの表示 plt.show()
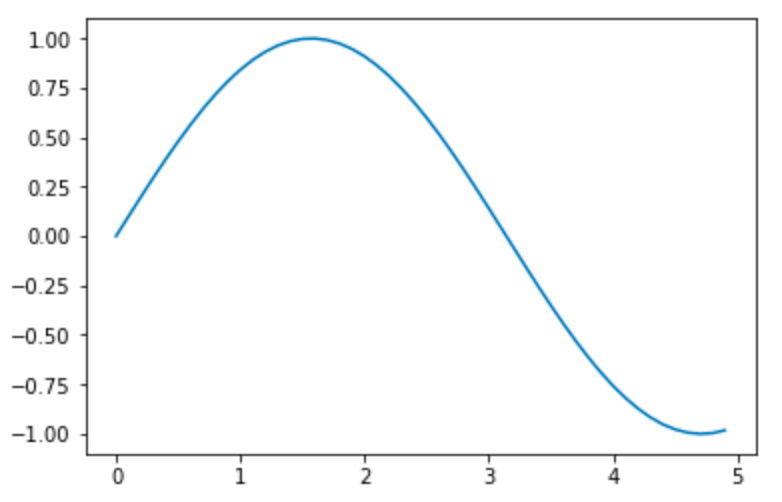
plt()の基本オプション
項目 | オプション | 説明(具体例) |
ラベル | label | グラフを表すラベル(凡例) |
色 | color | red, green, blue, cyan, magenta, yellow, black |
太さ | linewidth | 整数値(大きいほど太い) |
線の種類 | linestyle | dashed, dottedなどで点線や破線を指定 |
マーカー | marker | “o”や”x”等で指定 |
matplotlibでラベル(凡例)を追加
グラフの凡例を追加するには、plt.plot()にラベルを追加し、plt.legend()でタイトルを表示します。
#データ生成 x = np.arange(0, 5, 0.1); y = np.sin(x) #描画(ラベルを追加) plt.plot(x, y, label="sin_x") #ラベルの表示 plt.legend() #プロットの表示 plt.show()
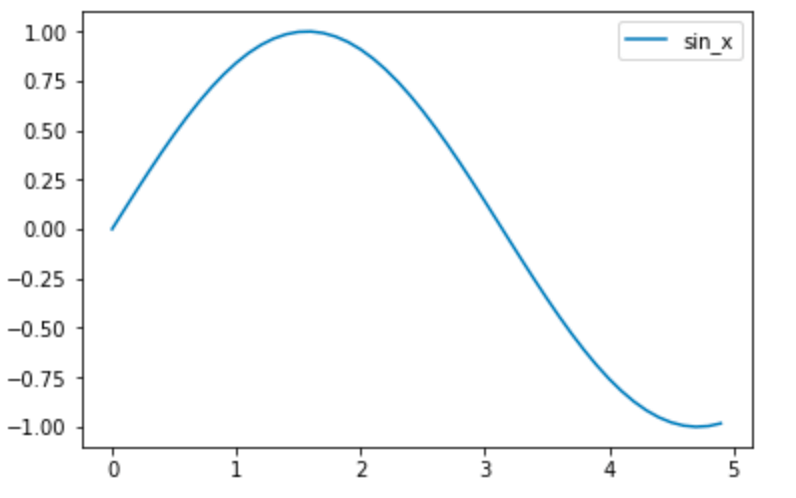
matplotlibで線の色や太さを変更
#描画 plt.plot(x, y, label="sin_x", linewidth=5, color="red")
線の太さを5に、色を赤に変更します。
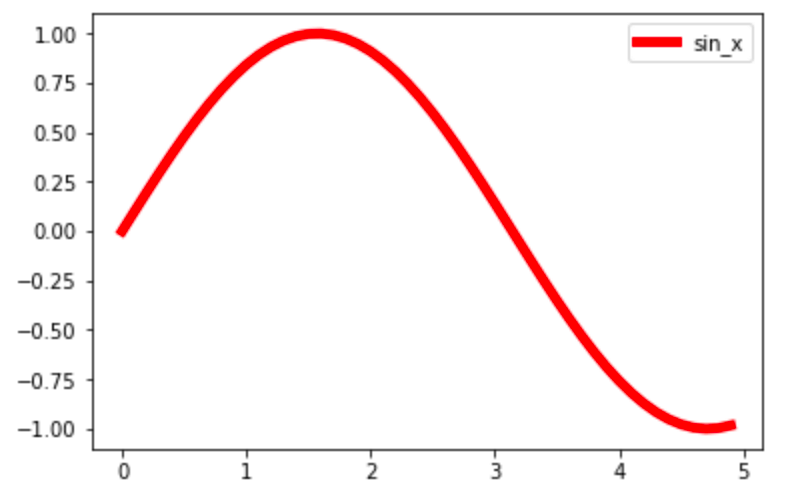
matplotlibで点線と破線を描画
#描画(破線と点線を追加) plt.plot(x, y, label="sin_x") plt.plot(x, y/2, label="sin_x / 2", linestyle="dashed") plt.plot(x, y/3, label="sin_x / 3", linestyle="dotted")
linestyleで線のスタイルを変更可能です。
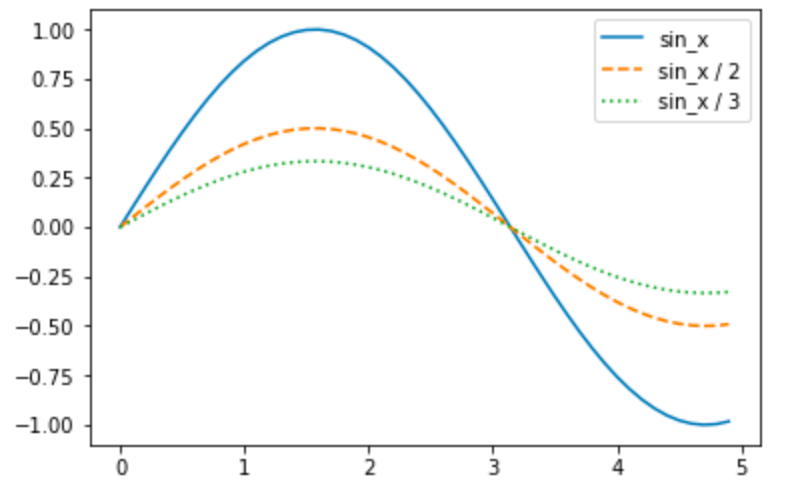
マーカーの追加
#描画(マーカを追加) plt.plot(x, y, label="sin_x", marker=".") plt.plot(x, y/2, label="sin_x / 2", marker="o") plt.plot(x, y/3, label="sin_x / 3", marker="x")
markerでマーカーを追加出来ます。
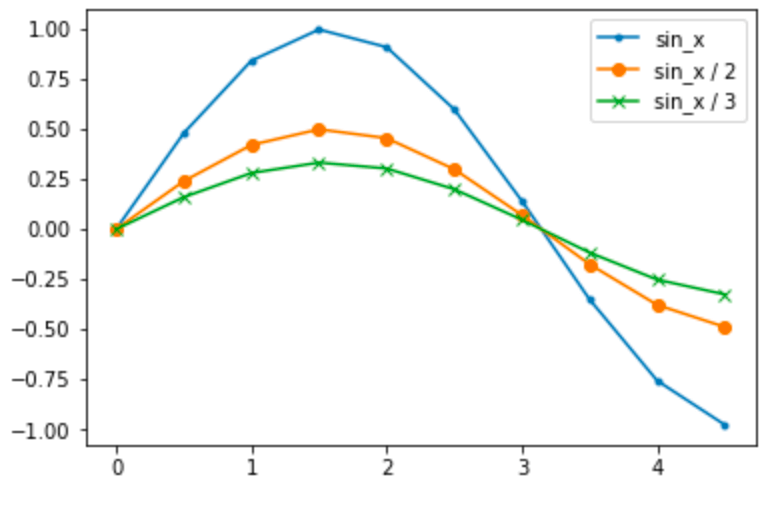
matplotlibでX軸・Y軸の名前を追加
#軸のタイトル plt.xlabel('x label') plt.ylabel('y label')
軸のタイトルを追加するには、plt.xlabelを使用します。
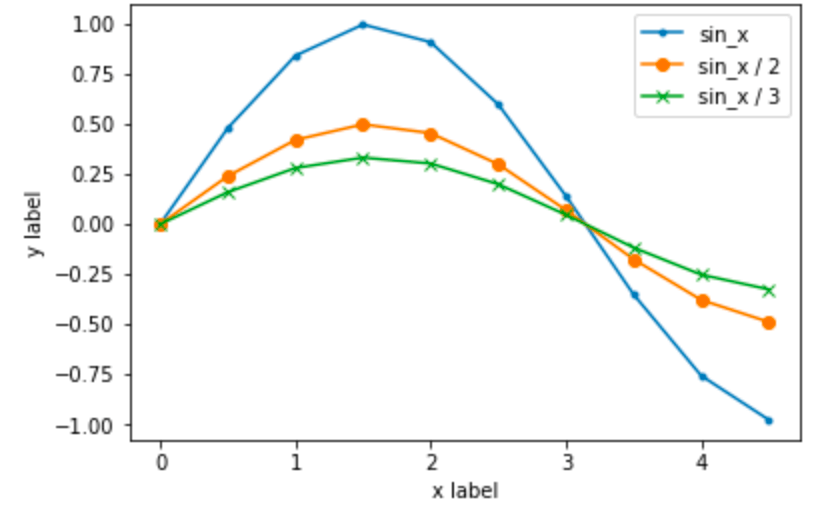